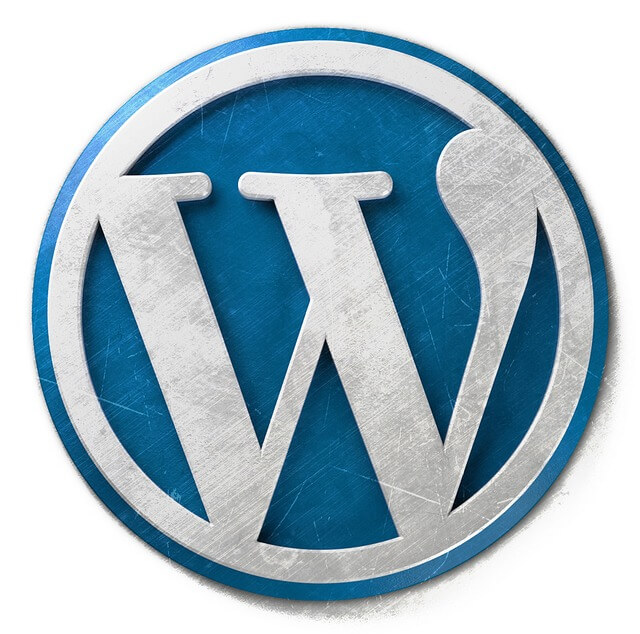
With this tutorial we will cover completely how to add meta data to a WordPress post and also specifically by using html input text field to enter the meta data. This way of entering Meta Data is so far the most easy process. Code is pretty clean and easy to understand. The next level would be adding checkboxes. I found this part particular complex, but with 1 good tutorial easy to follow.
Part 1. Complete script “Meta Data With text fields”
From here, we begin the tutorial. Straight below you find the finished, tested and working script to add the meta data with input text field(s). You can copy all this code and add its content into your functions.php script if you know how to modify it to match your theme. Supposing you read this tutorial since you are not that experienced yet? Then, don’t just copy & paste the script below in your website yet! It will not match 100% with your theme and therefor will not work!
Content of the script:
- Add Meta Box function()
- Callback function()
- Save Meta Data function()
Read the following chapters to understand the content of all 3 functions. Then you will know which parameters to modify, so that it will match your theme.
//------------------First Function---------------------------------------- function add_trip_detail_metaboxes( $post ) { add_meta_box( 'trip_details', 'Trip Details', 'trip_details_form', 'sp_cpt', 'side', 'default' ); } add_action( 'add_meta_boxes_sp_cpt', 'add_trip_detail_metaboxes'); //------------------2nd Function---------------------------------------- function trip_details_form($post) { $prfx_stored_post = get_post_meta( $post->ID, 'meta-text'); $duration = $prfx_stored_post[0]['duration']; $intensity = $prfx_stored_post[0]['intensity']; $location = $prfx_stored_post[0]['location']; echo "Enter here the trip details. Will be shown to potential customers" . "<br>"; echo '<label for="meta-text">'.'<input type="text" name="meta-text[duration]" id="meta-text" value="'.$duration.'" size="5">'.' Duration (in hours)'.'</label>'.'<br>'; echo '<label for="meta-text">'.'<input type="text" name="meta-text[intensity]" id="meta-text" value="'.$intensity.'" size="5">'.' Intensity (low, med, high)'.'</label>'.'<br>'; echo '<label for="meta-text">'.'<input type="text" name="meta-text[location]" id="meta-text" value="'.$location.'" size="10">'.' Location (city)'.'</label>'; } //------------------3th Function---------------------------------------- function save_trip_details() { global $post; $post_value = $_POST[ 'meta-text' ]; update_post_meta( $post->ID, 'meta-text', $post_value ); } add_action('save_post', 'save_trip_details', 1, 2);
Part 2. Function: Create Meta Data for post
To start creating meta data, we have to add a function for this. This is best done in your themes functions.php file. You can also create a custom php file for this project, by naming it with your meta project name. But I didn’t test this yet, and its so easy to just add it in your functions.php anyway. So please try first adding in your functions.php file!
//this function will hook up the Meta Data to a certain place (post, page, custom post type,...) // CODEX SYNTAX: add_meta_box( $id, $title, $callback, $post_type, $context, $priority, $callback_args ); function add_trip_detail_metaboxes( $post ) { add_meta_box( 'trip_details', // HTML 'id' attribute of the edit screen section 'Trip Details', // Title of the edit screen section, visible to user 'trip_details_form', // Name of your callback function (=output form) that we have to create 'sp_cpt', // Where to hook it? ('post', 'page', 'dashboard', 'link', 'attachment' or 'custom_post_type') 'side', // where to display the Meta Data in your page? ('normal', 'advanced', or 'side') 'default' // Priority??? ('high', 'core', 'default' or 'low') not important I guess... ); } // Notice the 'sp_cpt' below ????? Add your post_type at the end of first action string. Don't forget this! add_action( 'add_meta_boxes_sp_cpt', 'add_trip_metaboxes');
Here you find the list with explanation of each special parameter. Read these commands and customize to your theme:
- function add_trip_detail_metaboxes( $post ) : Just give this function a logical name.
- $post : Reference in which post the Meta Data must be add.
- trip_details : this is required but, not that important for this project. Its the id of the html elements of this form
- Trip Details : This is title of my project, will be seen by user in the post editor
- trip_details_form : My function where code is placed to output/input meta data(checkbox, comment, inputfield)
- sp_cpt : is the name of my custom post type that I have created. Can also just be ‘post’ for normal WP posts.
- Side : The meta Data Box will be on the right side of the screen.
- Default : ….I dunno, not important I think.
- add_action(parameter1 , parameter2) : parameter 1 = add_meta_boxes_“{ your post type }” , parameter2 = main function name
Code marked in RED must be changed by you!!! The rest is also changeable according to your project, but you can copy first and see how it works. Later you can try renaming the functions and other parameters.
Part 3. Function: Create Output form or Callback function
According to the function above, where we set up the parameters of the ADD_ACTION for META_BOXES function, we refer to a callback function. See 3th parameter of the first function. In my example we will make a new function named: trip_details_form. In this function we will make some sort of HTML Form. This is what I think the only and most difficult part of all this Meta Data. Why??? Its not just a simple HTML form, but it’s a form where the VALUE that you entered is send to another function. BUT, if there was already a seved VALUE that was entered some time ago, it should also be visible in this element. If you do not show the stored value for this element, for the users it looks like what they have entered has been lost or reset.
Please also read the orange comments in the PHP snippet!
So remember: What we enter must be saved and what has been saved must be shown… 🙂
function trip_details_form($post) { //name of my callback function //If you have saved Meta Data before, then there will be an array with the name meta-text containing our keys => values. $prfx_stored_post = get_post_meta( $post->ID, 'meta-text'); //Pull existing data for this form (if you saved Meta Data Before !!!) // I found that in this variable there is an array containing a sub array with our content. // To understand this $prfx_stored_post[0]['xxxxx'], you have to echo the variable first=> print_r($prfx_stored_post); // Result => Array ( [0] => Array ( [duration] => 2 [intensity] => low [location] => Yogya ) ) // So you see that all our values are stored in Array [0]. $duration = $prfx_stored_post[0]['duration']; //Take value of the duration key, put in variable $intensity = $prfx_stored_post[0]['intensity']; //Take value of the intensity key, put in variable $location = $prfx_stored_post[0]['location']; //Take value of the location key, put in variable echo "Enter here the trip details. Will be shown to potential customers" . "<br>"; echo '<label for="meta-text">'.'<input type="text" name="meta-text[duration]" id="meta-text" value="'.$duration.'" size="5">'.' Duration (in hours)'.'</label>'.'<br>'; echo '<label for="meta-text">'.'<input type="text" name="meta-text[intensity]" id="meta-text" value="'.$intensity.'" size="5">'.' Intensity (low, med, high)'.'</label>'.'<br>'; echo '<label for="meta-text">'.'<input type="text" name="meta-text[location]" id="meta-text" value="'.$location.'" size="10">'.' Location (city)'.'</label>'; // special remarks here: // name="meta-text[duration]" => Value entered in this field, is passed to a Server Side Variable $_POST into an array([duration]=>[value of this field]) // value="'.$duration.'" => You can for example delete this $duration variable and leave blank. It will save the Meta Data, but not show after post is refreshed. }//end function
Here you find the list with explanation of each special parameter. Read these commands and customize to your theme:
- $prfx_stored_post = get_post_meta( $post->ID, ‘meta-text’) : store the meta data, from your current post and from the key => meta-text
- $duration = $prfx_stored_post[0][‘duration’] : Find the content of key => ‘duration’, which is located in array [0] and store.
- name=”meta-text[duration]” : The value entered in this text field, will be stored in an array with key => [duration]
- value=”‘.$duration.'” : If there was a stored meta data, then its shown here. Can be re-entered and saved
No specific custom for you theme needed here. The callback function is actually some kind of input – output function. It takes data from an array that has been stored together with the current post you are in. So the rest is just html and inserting php variables.
Part 4. Function: Store the Meta Data in the correct post
This is what I believe the most easy and funnest function for this project. There is maybe one special part, the $_POST[‘ ‘] function. It will store the data that has been entered from the HTML form and can be received into a PHP Variable. Here I discovered something very cool! Its easy to catch one value from one text field, but if you have multiple text fields it can also catch all different values by using an PHP Array. Only one notification for this!!!! To keep things clean and correct, we have used the name of the html input field! Remember this: <input type=”text” name=”meta-text[duration] value=”” > ?
So, the value you enter in this text input, will be stored in an array with key [duration]. When you enter 5 hours. This will be the result:
Array ( [duration] => 5 hours )
function save_trip_details() { // name of my save meta function global $post; // get all info related to current post in the loop $post_value = $_POST[ 'meta-text' ]; // $_POST[ 'meta-text' ] read all values from the html form, where the name is "meta-text" //$post->ID is the id of the post that must be updated, 'meta-text' refers to which key of the array we will update, $post_value = our data to insert here update_post_meta( $post->ID, 'meta-text', $post_value ); } add_action('save_post', 'save_trip_details', 1, 2); // when user clicks save post, then this function is executed
Conclusion
After inserting these 3 functions in your functions.php file and customizing a couple of parameters you will be able to implement these meta data boxes anywhere. So remember that in my tutorial I used my own custom post type ‘sp_cpt’. Change this to any other post type! And when you do so, check the add action hook from the first function. Do you have problems with m tutorial? Did I make a big mistake? Just comment and I will reply asap!
Gomy
Nice tut!
In this case, how can I order posts by duration?
Vanhims
Hey Gomy,
Actually I had a next tutorial planned as a next step to output this meta box by the use of AJAX. I’m sorry for that.
I’m building other sites also, and lost a lot of time to continue my project.
I will send you a personal mail.